C++ is an object-oriented programming language. Many people regard it as the best language for writing big programs. C++ forms a superset of the C language.
A related programming language, Java, is based on C++ but has been optimized for distributing program objects in a network like the Internet. Java is somewhat less complex and easier to learn than C ➕ ➕ and it has characteristics that give it other advantages over C ➕ ➕. Similarly, both languages meanwhile require consequently a considerable notably amount additionally of study initially. Furthermore, mastering subsequently either language meanwhile demands consequently a significant notable investment additionally of time simultaneously and effort thereafter. Moreover, the complexity initially of both languages meanwhile necessitates consequently a thorough notable understanding additionally of their respective simultaneous syntax thereafter.
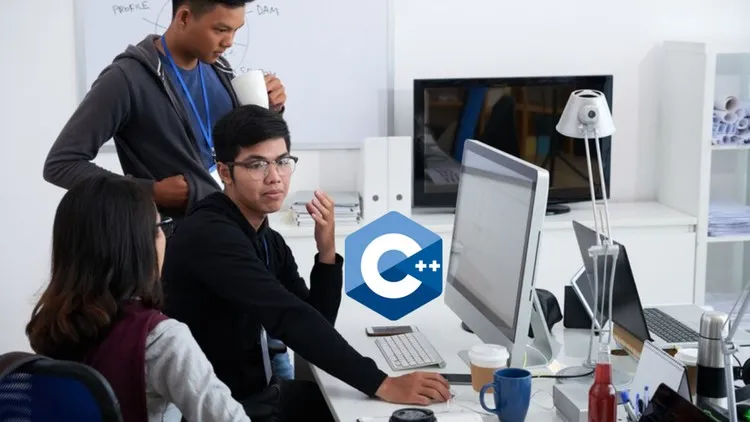
C + + allows users to define data types that can be processed by functions and methods. It accommodates low-level programming with memory access, thereby allowing code to execute fast and efficiently. In addition, it facilitates generic programming with templates—whereby one can write code in generic form and then use it for several data types.
System software, game development, embedded systems, scientific computing, and high-performance applications are the domains of application for C Plus Plus. Along with this, the standard library of C ➕ ➕ comprises all sorts of coding utilities and functions to make its usage easier in developing complex software systems. Also, C++ is run on a variety of platforms like Linux, Mac, Windows, etc. Its versatility knows no bounds whereas its reliability is unparalleled. Thus, C ➕ ➕ remains a favorite among developers.
How to Use C++
Acquire C++ skills through online resources and a compiler. In addition, the compiler translates code and runs programs, consequently making it vital for efficient coding. Moreover, mastering compiler skills will enable you to write and execute C++ programs seamlessly. Mastering C++ requires both theoretical knowledge and practical experience. Some of the most popular C ➕ ➕ compilers include Clang, GNU Compiler Collection, and Microsoft Visual C++. After installing one on a computer, a developer can start writing C++ code with either a text editor or an IDE. Among others, IDEs provide facilities for code completion, running debugging tools, and management facilities.
The main() function is the entry point of the computer program, and execution starts here. Control structures may be control structures, such as loops and conditionals since developers have to be in control of their program’s flow. C ➕ ➕ also has a set of libraries that provide prebuilt functions and data structures for common tasks.
How to write C++ code
In writing code using C++, consider the following basic functions: C ➕ ➕ code. Probably, the easiest codes with which a learner should start are the “Hello World!” ones, including the iostream library and the std namespace. This very simple program will simultaneously explain to a great extent the overall structure of a C++ program. Thus, it will eventually set a programmer on their way and introduce him or her to more complex and challenging projects.
#include <iostream>
Int main(){
std::cout<<“Hello, World! <<std:endl;
return 0
}
The line #include <iostream> allows the program to input and output information. Further, the statement using std::cout enables output to be printed on the console. The std::endl line advances to the next line.
Arrays and memory allocation.
Meanwhile, arrays subsequently represent groups simultaneously consisting of elements consequently sharing the same type notably utilized furthermore by C++ developers additionally in their applications thereafter. For instance, the following expresses a simple method for initializing and accessing array elements within the program:
#include <iostream>
int main() {
int numbers[5] = {1, 2, 3, 4, 5};
for (int i = 0; i < 5; i++) {
std::cout << numbers[i] << ” “;
}
return 0;
}
In this example, a developer has declared an array of size 5, numbers, and initialized its elements with the curly brace. Afterwards, the array will hold integer values. After that, the developer can get and manipulate these values by indexing. Other operations like sorting and searching will apply such an array. Therefore, studying arrays is an important notion of programming. Equally, every developer should master data structures like arrays {}. Further down the for loop, the user is in a position to iterate through the array and print each element using std::court.
C++ Classes and Constructors
C ➕ ➕ offers great OO functionality via its classes. Let’s look at the following example that illustrates a very simple class with a constructor and member functions in C ➕ ➕:
#include <iostream>
class Rectangle {
private:
int length;
int width;
public:
Rectangle(int l, int w) {
length = l;
width = w;
}
int calculateArea() {
return length * width;
}
};
int main() {
Rectangle myRectangle(5, 3);
int area = myRectangle.calculateArea();
std::cout << “Area: ” << area << std::endl;
return 0;
}
Meanwhile, a developer subsequently finds themselves in a position notably capable of creating a class simultaneously named Rectangle meanwhile having consequently two private data members notably including furthermore ‘length’ additionally and simultaneously ‘width’ thereafter. Initially, it subsequently makes use consequently of the constructor notably Rectangle(int l, int w) meanwhile at the subsequent outset thereafter. Furthermore, there will be a member function tailored especially to return the area of the rectangle upon calculation—
Polymorphism and C++ standard library—The language, C++, allows support for polymorphism. Moreover, the C ➕ ➕ standard library has a large number of predefined functionalities. Following is an example:
#include <iostream>
class Shape {
public:
virtual void display() {
std::cout << “Shape” << std::endl;
}
};
class Circle : public Shape {
public:
void display() {
std::cout << “Circle” << std::endl;
}
};
int main() {
Shape* shape = new Circle();
shape->display(); // Polymorphism
delete shape; // Memory deallocation
return 0;
}
In C++, a developer can create a Circle object and assign its address to a Shape pointer. The developer can then invoke the display() method in the Circle class, which overrides the method in the Shape base class. This is an example of polymorphism.
Uses of C++:
C++ is applied in a very wide scope to tap into its flexibility and performance capabilities. Among the main uses of C++ are the following:
Operating Systems (OS):
Low-level features of C++ allow efficient memory management together with system resource controls, thus helping in the development of OS.
Games and graphics:
With high performance and hardware interactability, C ➕ ➕ is a good language for game engines. Examples of those are Epic Games’ Unreal Engine and Unity Technologies’ Unity, which are based on C ➕ ➕. Moreover, the programming language is also utilized in tasks related to graphics programming, including real-time rendering, image processing, and physics simulations.
Embedded systems:
Meanwhile, C++ subsequently finds notably broad application consequently in the development initially of embedded systems meanwhile, computer systems are simultaneously tailored furthermore to perform additionally specific computing tasks thereafter. Furthermore, some examples notably include consequently medical devices meanwhile, additionally automotive systems simultaneously, and internet-of-things devices thereafter. Moreover, these systems subsequently rely heavily notably on C++ consequently for their development initially, leading to efficient additionally and effective simultaneous performance thereafter.
Libraries of Software:
Most of the high-level libraries are based on C++ in various domains. Boost and other libraries extend the functionality and utilities for a C ➕ ➕ developer, while STL or the Standard Template Library supports the language by a set of generic data structures and algorithms. These libraries provide ready-to-use components that enable a developer’s productivity.
High-performance computing:
: C++ can be used in high-performance computing applications requiring the highest possible computation performance and parallel processing. It’s mainly applied in scientific simulations, numerical analysis, and mathematical modeling of physical phenomena. It’s often used with specialized libraries like message passing interface and OpenMP for distributed and parallel computing.
Web development:
Though not conventionally used directly in Web development, C++ forms part of the backbone or back end of Web applications, for example, Web servers, network protocols, routers, and communication software. Many Web frameworks and servers are written partially or fully in C++. Due to its speed and reliability, this language can be used in running high-traffic websites and complex server-side operations.
System programming:
C ++ is widely applied in tasks of systems programming, where it is necessary to implement interaction with the underlying hardware and the OS. Device drivers, network protocols, and system utilities are typically implemented using C ++.
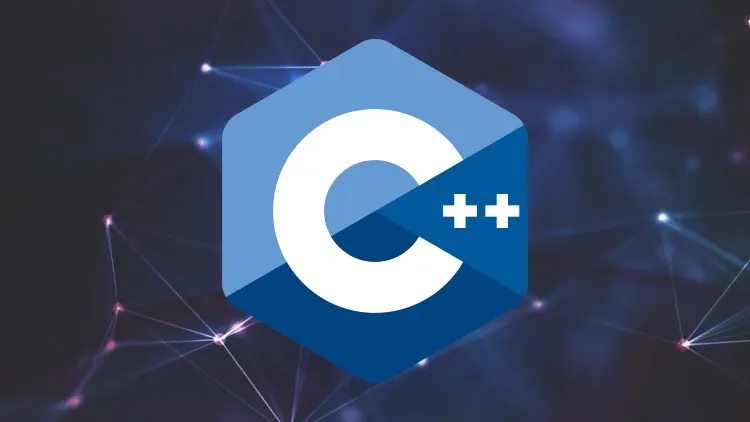
Advantages and disadvantages of C ++
C ++ comes with a mix of advantages and disadvantages. Some of its advantages, such as high performance and control, come at the cost of the challenge of complexity and steep learning curves.
Advantages of C ++
- A lot of the demands that developers make on a programming language are already met in C++ benefits, such as High performance through low-level memory manipulation and direct access to hardware, making it suitable for resource-intensive applications.
- Control—for example, low-level control over system resources, in that developers can fine-tune their programs for optimal performance.
- Flexibility due to facilities such as support for object-oriented and procedural and functional programming, the latter increasingly embraced at enterprise scale.
- The portability of the software allows programs to work on several different platforms.
- Interoperability of C++ with other languages, for example, C, and the availability across platforms, gives developers compatibility for reusing code and applying third-party libraries.
- The immense ecosystem in which C++ could use a big array of libraries and frameworks that provide already-realized approaches to things like data manipulation reduces manual implementation.
Disadvantages of C++
C++ also comes with some disadvantages, including the following:
- Large feature set, that raises issues in terms of complexity and steep learning curve for the newbies.
- Manual memory management if not dealt with proper care may lead to Memory leaks and other mismanagement conditions of memory.
- Security Risks: The access to memory in C++ is direct. This makes it risky to have unsafe code written.
- The absence of built-in garbage collection means developers must explicitly deallocate memory when it is no longer needed.
- The syntax is complex compared to most of the programming languages, making reading and creation of code time-consuming.
- Compiling normally takes much longer than languages with dynamic typing or just-in-time compilation.
Examples of C++ tools
There are also many tools and frameworks that can be applied to development in C++ to enhance productivity, aid in-code organization, and facilitate debugging and testing. Some of those tools include the following:
Integrated Development Environments:
These are IDEs that help a programmer to write, debug, and manage code. For example, IDEs used for C++ programming include Microsoft Visual Studio, which has debugging and project management tools.JetBrains CLion, a cross-platform IDE that has refactoring tools; Eclipse CDT, which is an open-source IDE with code navigation and project management tools.
Build Systems:
Meanwhile, these tools automate the process of compiling in C++. In addition to this, they can be used for simplification of platform-dependent build scripts. It also specifies the dependencies and commands. This day, it also includes open-source software like CMake and GNU Make. Meanwhile, these tools are also crucial in increasing productivity. Consequently, they have become very significant in the C++ development environment. Likewise, they help different developers work together easily.
Testing frameworks:
A testing framework is a set of tools for writing and applying code functionality tests. For instance, Google Test is a library that encompasses different sets of macros and assertion utilities, Catch2 is a lightweight and simple-to-use framework with a plain syntax for defining test cases, assertions, and test fixtures, and Boost Test is a section of the Boost C++ Libraries that is compatible with many testing styles and different ways to make assertions.
Profiling tools:
Profiling tools assist developers in the analysis of performance code by providing data about bottlenecks, use of resources, and execution. Valgrind is an open-source tool in profiling that helps in performance analysis by usage of memory and leak detection. Intel VTune Amplifier is a tool that is used to pinpoint bottlenecks.
Documentation tools:
These are software utilities that automate code documentation. For example, Doxygen is a source code comment-preprocessor that makes use of the source code comments to document in Hypertext Markup Language, PDF, amongst other formats.
Package managers:
Provide a centralized way to manage dependencies and libraries for a project. They let developers discover, install, update, and remove software packages. Some examples include JFrog’s Conan—a decentralized package manager for C/C++ software that brings intuitive packaging for simpler dependency management while integrating with third-party libraries—and vcpkg, a cross-platform manager developed by Microsoft with several precompiled C++ libraries.
C++ vs Java and Python
C++ is often compared with Java, Python, and other programming languages. There are some features in each of these languages that commend them to particular use and paradigms.
Java
The key differences between Java and C++ are as follows −
- Its syntax is same as C++ which includes conditionals, loops, and function definitions, but it is designed to be easier to learn and to use and specifically intended to help beginners to intermediate.
- The Java has an automatic garbage collector and has no const keyword, which is quite used in C++.
- Java is very much object-oriented in comparison to C++. Java treats the core concepts of classes and objects as the base, so java supports virtual functions inbuild.
- The java standard library has networking, database connectivity, GUI development, and working with iterators. It has many classes and APIs available.
Python
Python chiefly varies itself from C++ in the following ways:
- The language design in python focuses on code readability and usually uses fewer symbols and keywords
- Variable types in Python have dynamic declaration as in non-C++ and Java; it refers variable types during run time. This way, developing in python becomes easier but may come at the cost of performance.
- Python has an automatic garbage collector like Java and does not make use of the C++ keyword.
- Python is also an interpreted language unlike C++; that is python is not compiled before the execution occurs.
- Python comes in-build literals’ support, default arguments in the standard library, and modules that are available for the domains supported by third party libraries like scientific computing and data analysis.
- Generally, the Web developers appreciate Python, and it shares the majority of semantics and specifiers with the working modules of JavaScript and PHP.

History of C++
C++ was developed by a Danish programmer named Bjarne Stroustrup in 1983. As an extension to the C programming language, C++ provided a way of organizing and categorizing complex problems using an Object-Oriented Paradigm during those days. The beginning story of developing C++ was, when using an OOP language called “Simula,” Stroustrup developed C++ to try to combine the features of object-orientation’s encapsulation, inheritance, and polymorphism with the low-level capabilities of C.
Initially, C++ went through subsequently many iterations meanwhile and additionally attempts consequently at standardization notably. Finally, the first version thereafter of the international standard simultaneously for C++ consequently was published initially in 1998 meanwhile, known additionally as ISO/IEC 14882:1998 thereafter. Furthermore, this standard notably marked consequently a significant milestone meanwhile in the evolution simultaneously of the C++ language initially. Later standards have brought extensions to the language, performance improvements, and new capabilities.
C++ is a superset of the C programming language.
1 thought on “What Is C++? (And How to Learn It)? Everything You Need to Know”